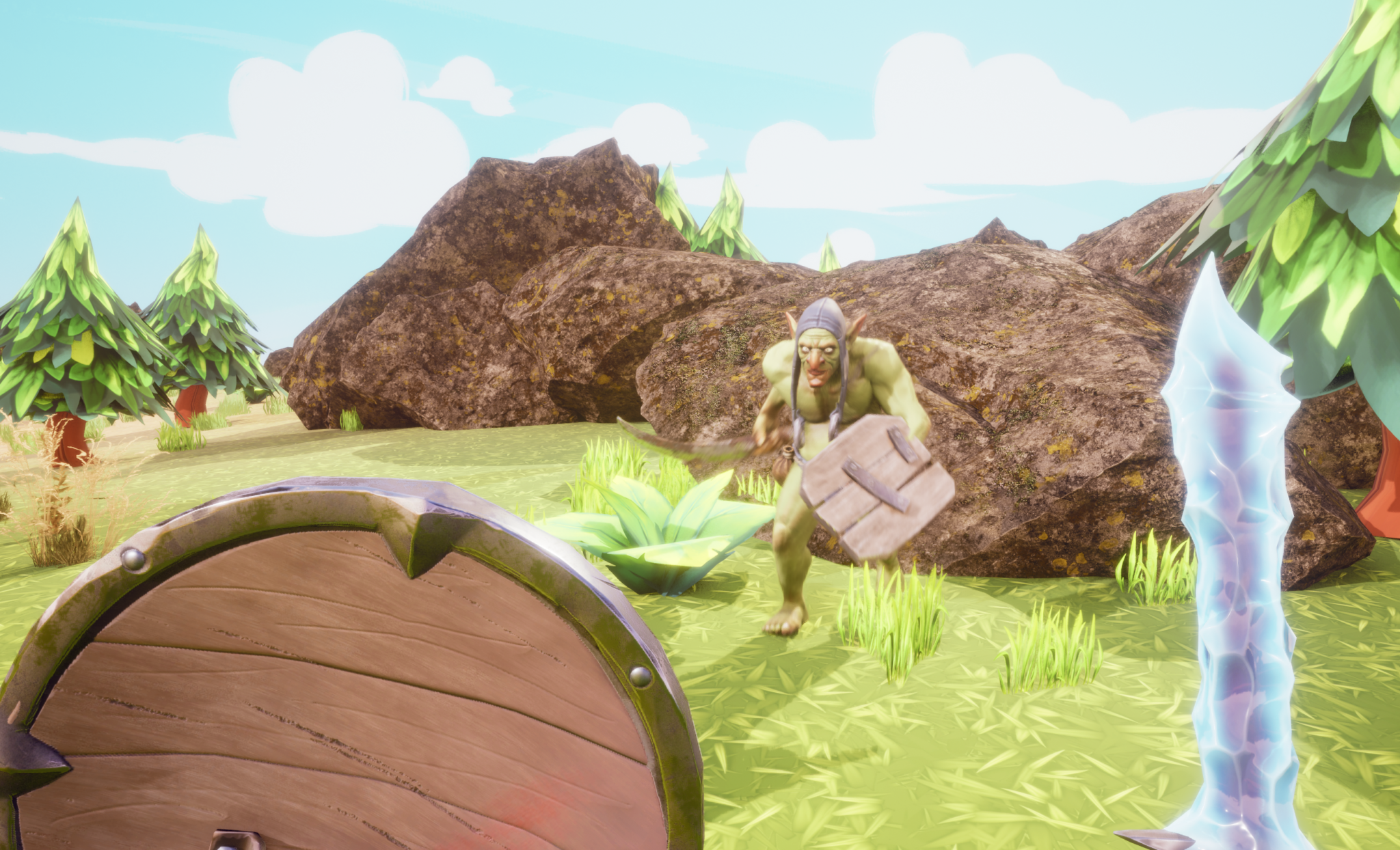
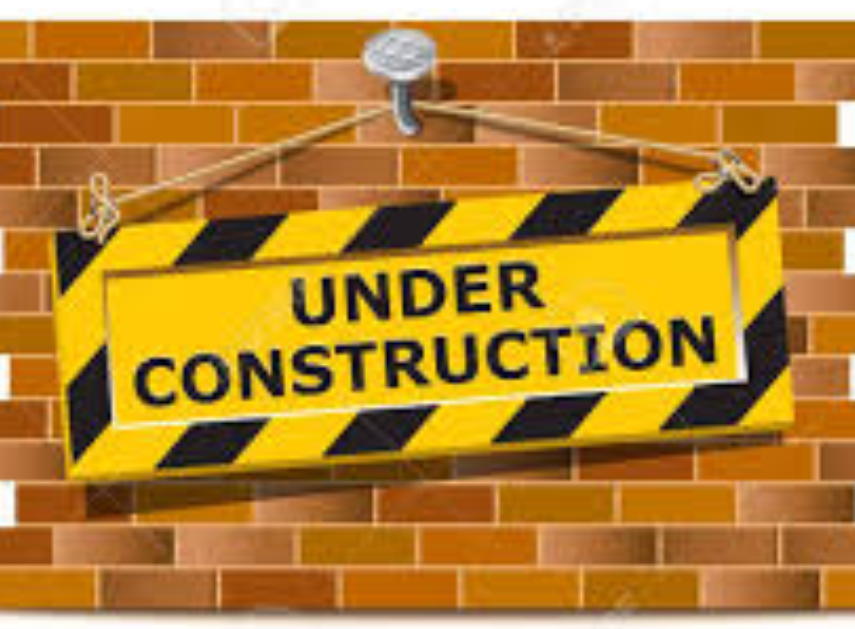
Requirements
Let’s start off by looking at the requirements of the system we are trying to build.
- Character movement: The player character should be able to move around the environment and dodge attacks.
- Basic attacks: The player character should have a basic attack that can be used repeatedly.
- Special attacks: The player character should have a variety of special attacks that can be used in different situations. These attacks can be tied to specific buttons or key combinations.
- Blocking and parrying: The player character should be able to block or parry incoming attacks to reduce damage.
- Enemy AI: Enemies should have their own AI that determines their behavior in combat. This can include attacking the player character, dodging attacks, and using special abilities.
- Health and damage: The player character and enemies should have health bars that decrease as they take damage. The amount of damage dealt can depend on various factors, such as the type of attack used and the enemy’s armor.
- Loot and rewards: Defeating enemies can reward the player with loot, such as weapons, armor, and items.
- User interface: The user interface should display important information, such as health bars, enemy names, and the player’s current abilities.
Let’s examine a simple example, some basic combat support with collision detection in a 2d game.
// Define a class for the player character
class Player {
public:
float x, y; // position of the player
float width, height; // dimensions of the player
float speed; // movement speed of the player
bool isAttacking; // whether the player is currently attacking
// other properties and methods as needed
};
// Define a class for the enemy character
class Enemy {
public:
float x, y; // position of the enemy
float width, height; // dimensions of the enemy
float speed; // movement speed of the enemy
bool isAlive; // whether the enemy is alive
// other properties and methods as needed
};
// Define a function to check for collision between two rectangles
bool isColliding(float x1, float y1, float w1, float h1, float x2, float y2, float w2, float h2) {
return (x1 < x2 + w2 && x1 + w1 > x2 && y1 < y2 + h2 && y1 + h1 > y2);
}
// Define a function to handle combat between the player and an enemy
void handleCombat(Player& player, Enemy& enemy) {
if (player.isAttacking && isColliding(player.x, player.y, player.width, player.height, enemy.x, enemy.y, enemy.width, enemy.height)) {
// Player is attacking and colliding with the enemy, deal damage to the enemy
enemy.isAlive = false;
}
else if (isColliding(player.x, player.y, player.width, player.height, enemy.x, enemy.y, enemy.width, enemy.height)) {
// Player is not attacking but colliding with the enemy, take damage
// Reduce player's health or other appropriate action
}
}
// In the game loop, call the handleCombat function for each enemy
while (gameRunning) {
// Move player and enemies
// Handle input
// Update game state
for (int i = 0; i < numEnemies; i++) {
handleCombat(player, enemies[i]);
}
}